Intro
Since HTML5 was released, the hassle of using flash to add multimedia content was over. Now we can simply inject any video within the HTML5 itself. The HTML5 video player has the normal controls such as play/ pause buttons, the progress bar, the timer. It also has properties such as video type and its duration. Unfortunately, most automation testing tools DO NOT provide any control over the <Video> tag within HTML5.
In this article, we will be discussing a simple mechanism to automate a video player, extract the video’s info, and assert on its properties.
Why do we need to automate media?
If your web or mobile application has an audio or video that plays or pauses depending on a specific action that the user does, so you need simply to check if your media is working as expected according to the feature or scenario under test.
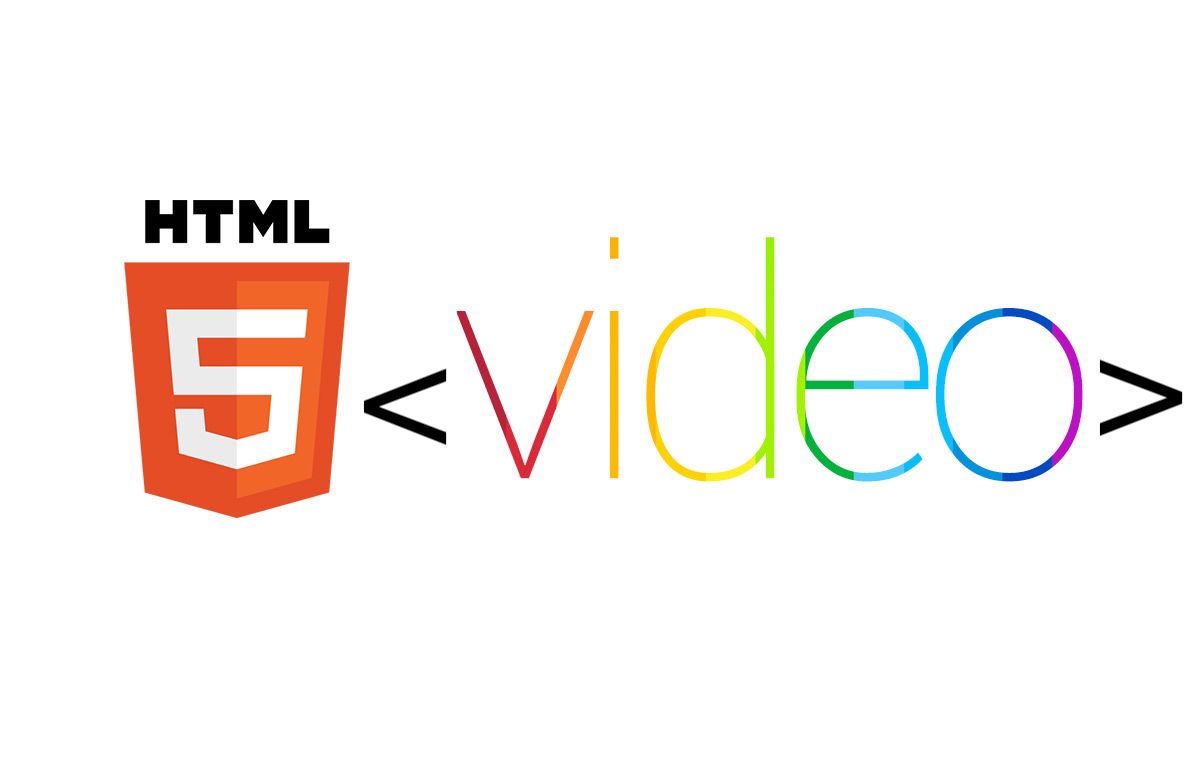
How it works?
It’s been said that whatever the automation environment or tools you’re using, you will not find built-in functions that can handle <Video> locators directly. As a result, you won’t be able to access the simplest controls within videos such as the PLAY (▷) button, the VOLUME controller, the PROGRESS BAR, the DURATION indicator … etc.
The only way to access these controls is by using JavaScript commands. JS has certain functions and properties that can deal with video controls inside <Video> tag. Some of these controls are as follows:
- play(): Function: plays the targeted video,
- pause(): Function: pauses the targeted video,
- paused: Property: returns TRUE if the video is paused,
- Duration: Property: returns video’s duration (in seconds),
- volume: Property: returns the sound volume controller,
- currentTime: Property: returns the current time that videos is played/ paused at (in seconds),
- …..
So, let’s list the exact steps you will follow in your automation script:
- You should wait until the metadata of your video is loaded, if the pre-load option of your video is not ‘auto’ (auto means that the whole video file will be loaded without waiting)
This can done by using onloadedmetadata event as following:
var vid = document.getElementById("myVideo");
vid.onloadedmetadata = function() {
alert("Metadata for video loaded");
};
2. After you select your video (by ID or any other selector) and waiting for it to load, you can use any supported HTML functions and attributes of dealing with media, you can find them here
3. The most important trick here is that your code should be handled by JavaScript executor because there are no direct functions to use for videos. Since we are dealing with some JS functions and attributes, we’re gonna open a shell that can execute JS commands with the browser’s console. Within this shell, we will be executing the functions and properties for the video, and retrieve any data that we may use to assert on.
4. Finally, you can use any attribute related to media. For example, currentTime attribute to set or get the current time of your played video:
var vid = document.getElementById("myVideo");
vid.currentTime = 5;
this will set the time of your playing video at 5 seconds, so if your video duration is 10 seconds, this command will move the progress bar of the video to the half of its duration.
Conclusion
There is a list of supported functions and attributes for dealing with media in HTML5, you can use all of them whatever the language you’re using in your script if you used the JavaScript executor, main checks you need will be doable like checking your video is paused or not and get its duration or check if it has controls or not but there is no method for reaching the full-screen button or download options of your video, so you can do the prime checks and make it simple.